isaac_resources
A very important table! As explained on the last page, every game resource is stored here: pills, runes, items, enemies, bosses...
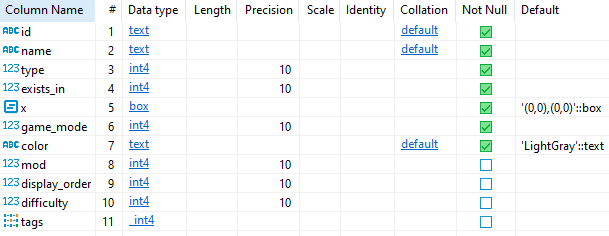
-
id
- Primary Key. The unique ID, roughly resembles the name of the resource. For example: "BasementOne", "CarrionQueen" or "AceOfClubs". All tables that refer to isaac resources use this ID! -
name
- the name of the resource, as it is displayed in-game like "Basement 1", "Carrion Queen" or "Ace of Clubs". -
type
- what type of resource it is:- Unspecified
- Boss
- Playable Character
- Curse
- Other Event
- Floor
- Item
- Item Source (example: Item Room)
- Pill
- Rune
- Tarot Card
- Enemy
- Transformation
- Trinket
- Character Reroll (example: D100)
- Other Consumable
-
exists_in
- In what version(s) of isaac does this resource exist?- Nowhere
- Every Isaac Version
- Vanilla (Flash) Exclusive
- Wrath of the Lamb (Flash) Expansion Exclusive
- Community Remix Exclusive
- Rebirth Exclusive
- Afterbirth Exclusive
- Antibirth Exclusive
- Afterbirth Plus Exclusive
- Repentance Exclusive
- Booster Pack 1 Exclusive
- Booster Pack 2 Exclusive
- Booster Pack 3 Exclusive
- Booster Pack 4 Exclusive
- Booster Pack 5 Exclusive
- Vanilla (Flash) and all versions going forward
- Wrath of the Lamb (Flash) and all versions going forward
- CommunityRemix and all versions going forward
- Rebirth and all versions going forward
- Afterbirth and all versions going forward
- Anitbirth and all versions going forward
- Afterbirth Plus and all versions going forward
- Booster Pack 1 and all versions going forward
- Booster Pack 2 and all versions going forward
- Booster Pack 3 and all versions going forward
- Booster Pack 4 and all versions going forward
- Booster Pack 5 and all versions going forward
- Repentance and all versions going forward
- Unspecified
- Antibirth, Repentance and all versions after Repentance
- Community Remix and Antibirth
- Community Remix, Antibirth, Repentance and all versions after Repentance
- Community Remix, Antibirth, Afterbirth and all versions after Afterbirth
- Vanilla and Wrath of the Lamb (Flash Versions Exclusive)
- Community Remix, Rebirth and all versions after Rebirth
-
x
- the CSS coordinates for this resource in this giant ass image. Probably not too useful outside of this website -
game_mode
- In which game mode does this resource exist? Almost everything has, as you might imagine, set this to "All Modes".- All Modes
- Normal Only
- Hard Only
- Greed Mode Only
- Greedier Mode Only
- Special Challenge
- Special Seed
- Unspecified
- Hard and Normal Mode
- Community Challenge Specific
- Greed and Greedier Mode
-
color
- an appropriate color for the resource. Again, probably not too useful outside of this webpage. -
mod
- this references the primary key of themods
table if the resource was introduced by a mod. -
display_order
- a simple integer used for sorting resources in a custom way. -
difficulty
- unused for now, but could be used to assign a difficulty rating to bosses for example. -
tags
- resources are tagges as "Poisons Enemies", "Is a spacebar item" or "appears on Basement 1", and so on. This integer array stores all flags that were assigned to the resource. The list of tags is quite big (250+), refer to this page for a full list
Query Examples:
Find all bosses:
select * from isaac_resources where type = 1;
Find everything that starts with 'A':
select name from isaac_resources where lower(name) like 'a%';
Find all resources that are tagged as spacebar item:
Tags are saved as integer array. Use the '@>' operator to see if the integer array contains a specific tag.
select name from isaac_resources where tags @> array[139];
Find everything that comes from a mod:
select name, mod from isaac_resources where mod is not null;
Same, but joining the mods table for mod names:
select isaac_resources.name, mods.name
from isaac_resources
join mods on mods.id = isaac_resources.mod
where isaac_resources.mod is not null;
mods
Very simple. Only contains a primary key and the name of the mod.

-
id
- Primary key. -
name
- the name of the mod.
mod_url
Saves external links for mods, for example to the steam workshop page or a wiki.

-
id
- Primary Key -
url
- the link URL -
name
- the link text -
mod
- references the ID of the mod this link belongs to
Query Examples:
Get all links for the Antibirth mod:
select mods.name, mod_url.name, mod_url.url
from mods
join mod_url on mod_url.mod = mods.id
where mods.name = 'Antibirth';
videos
Stores video data - everything except the timestamp at the end is directly pulled from the youtube API.
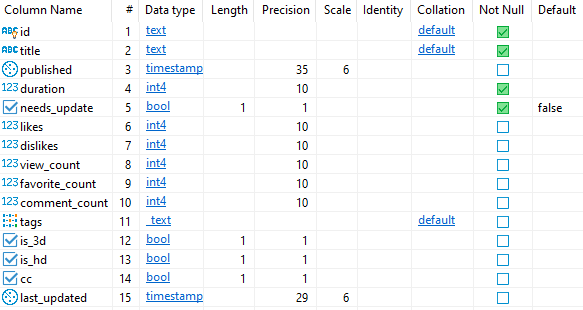
-
id
- Primary Key. The same 11-character ID youtube uses for videos. Everything in the database that references a video will refer to this 11-character ID! -
title
- the video title, for example: "The Binding of Isaac: AFTERBIRTH+ - Northernlion Plays - Episode 186 [Trainwreck]" -
published
- the release date of the episode -
duration
- the video duration in seconds -
needs_update
- a flag that can be set if the episode needs to be updated. Can be ignored. -
likes
dislikes
view_count
favorite_count
comment_count
are exactly what they say -
tags
- the tags Northernlion added to the episode when he uploaded it -
is_3d
- pretty useless. indicates whether the video is in 3D or not. -
is_hd
- if the video is 720p and up. -
cc
- if the video has subtitles -
last_updated
- timestamp indicating when the data was last updated from the youtube API
Query Examples
Search the video title for 'Trainwreck'
select * from videos where lower(title) like '%trainwreck%';
Search for videos that are longer than an hour
select * from videos where duration > 3600;
Find the episode with the most likes
select title from videos where likes = (select max(likes) from videos);
video_submissions
This is what you call 'an isaac episode'. Saves some metadata every time a user submits an episode that ties everything together.

-
id
- Primary Key. Everything that belongs to this submission will reference this ID! -
video
- the 11-character ID of the video. See "videos" table. -
s_type
- type of submission - for internal use. Can be:- Lost submission, no data is available for this one.
- Old submission, misses data old versions of this website didn't track (like runes, pills, reroll transformations and so on)
- New submission, was submitted since this version of the website came out.
- Unknown
-
latest
- episodes can be submitted multiple times by multiple users. This flag indicates if it's the most recent submission for this episode.
Query Examples
Find all submissions for the first isaac episode
select * from video_submissions where video = 'c5PLC6nmOO4';
Find the most recent submission for the first isaac episode.
Make sure to always include the "latest" flag when querying submissions, because episodes can be submitted multiple times by multiple users.
Otherwise you will most likely get duplicate entries or submissions with missing data.
select * from video_submissions where video = 'c5PLC6nmOO4' and latest = true;
played_characters
Every 'isaac episode' described in the table above can have multiple played characters, so every entry in this table is what you call 'a run'. Most of the time there will only be a single character per submission, but sometimes there can be quite a few (for example episodes where Northernlion plays as Keeper or The Lost).
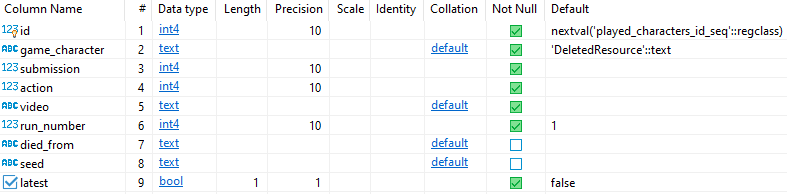
-
id
- Primary Key. Everything that happened to this character will reference this ID! -
game_character
- the ID of the isaac_resource (see first section of this page) that resembles this character. -
submission
- the ID of the submission this character belonged to -
action
- a counter that counts up (+1) for every action that was taken by this character. Can be used to sort all actions chronologically. -
video
- the 11-character ID of the video. See "videos" table. -
run_number
- as was already discussed, a submission can have multiple runs. This is a counter that starts at 1 and is incremented for every run. Example:- Run 1: game_character = Isaac; run_number = 1
- Run 2: game_character = Maggie; run_number = 2
-
died_from
- If the character survived the run, this will be null. If not, this will be the ID of the isaac_resource of the enemy who killed the character. -
seed
- the seed for the run. If Northernlion didn't show the seed, this will be null. -
latest
- same as above: true if this is part of the newest submission for this episode.
Query Examples
Finding all characters and deaths from the infamous "Trainwreck" episode.
As described in the last section, always check for the "latest" data.
select game_character, died_from from played_characters where video = 'JfDnrW-pnXU' and latest = true;
Finding all characters NL killed by "Bombing Himself"
select game_character, died_from from played_characters where died_from = 'BombedHimself' and latest = true;
Same as above, but with video title and sorted by release date.
Because everything references the "video" table, you can get the video title with a simple join:
select videos.title, played_characters.game_character, played_characters.died_from
from played_characters
join videos on videos.id = played_characters.video
where played_characters.died_from = 'BombedHimself'
and played_characters.latest = true
order by videos.published desc;
Find all characters NL killed on a sunday:
select videos.title, played_characters.game_character, played_characters.died_from
from played_characters
join videos on videos.id = played_characters.video
where played_characters.died_from is not null
and played_characters.latest = true
and extract(DOW from videos.published) = 5;
played_floors
Saves all floors a "played_character" from the last section went through.
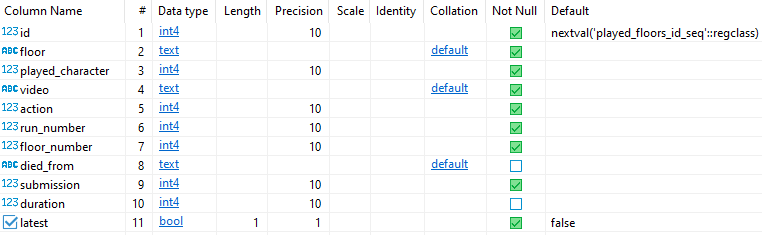
-
id
- Primary Key. All events that happened on this floor will reference this ID! -
floor
- the ID of the "isaac_resource" that resembles this floor. -
played_character
- the ID of the played_character from the above section who went through this floor -
video
- the 11-character ID of the video. See "videos" table. -
action
- same as above. a counter that counts up (+1) for every action that was taken by this character. Can be used to sort all actions chronologically. -
run_number
- same as above: a submission can have multiple runs. This is a counter that starts at 1 and is incremented for every run. Example:- Run 1: game_character = Isaac; run_number = 1
- Run 2: game_character = Maggie; run_number = 2
-
floor_number
- starts at 1 and increments for every floor after that. can be used to sort floors in the order they appeared in the run. -
died_from
- if the character died on this floor, the ID of the "isaac_resource" that killed the character will be here. Otherwise this will be null. -
submission
- the ID of the submission this floor belongs to. -
duration
- how long did NL stay on this floor in seconds? -
latest
- same as above: true if this is part of the newest submission for this episode.
Query Examples
Find all floors from the first isaac episode (in chronological order)
select * from played_floors where video = 'c5PLC6nmOO4' and latest = true order by floor_number;
Find all floors on which NL died by bombing himself
select * from played_floors where died_from = 'BombedHimself' and latest = true;
Find the floor that was visited the most
select floor, count(floor) as times_visited
from played_floors
group by floor
order by times_visited desc;
Find the floor Northernlion spent the most time on
select floor, duration
from played_floors
where latest = true
and duration = (select max(duration) from played_floors);
...including the video title
select played_floors.floor, played_floors.duration, videos.title
from played_floors
join videos on videos.id = played_floors.video
where played_floors.latest = true
and played_floors.duration = (select max(duration) from played_floors);
gameplay_events
All gameplay events. Probably the most interesting table of all.
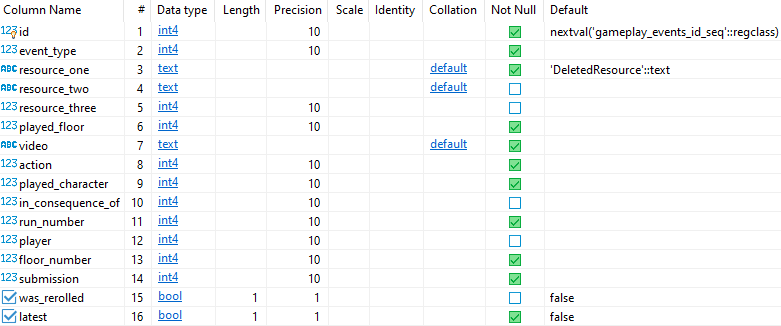
-
id
- Primary Key. -
event_type
- what type of event it was:- Unspecified (unused)
- Character Died
- Item Collected
- Down to the next Floor!
- Bossfight
- Pill used
- Tarot Card used
- Rune used
- Trinket taken
- Floor was Cursed
- Other Consumable used
- Transformation Complete
- Transformation Progress
- Last Floor (unused)
- Absorbed Item
- Character Reroll
- Won the run
- Lost the run
- Item Touched (picked up and put down again right away)
- Starting Trinket
- Clicker (changed character with the clicker)
- Reroll Transform (transformation that happened after rerolling the character)
- Respawn (respawning after death due to extra lives)
-
resource_one
- the first isaac_resource that was part of this event. For example:- item collected: this will be the ID of the item.
- transformation progress: this will be the ID of the item that triggered the transformation progress.
- pill used: this will be the ID of the pill.
-
resource_two
- the second isaac_resource that was part of this event (if needed). For example:- item collected: this will be the ID of the item source.
- transformation progress: this will the the ID of the transformation towards which progress was made
- pill used: NULL
-
resource_three
- integer that is only used for counting transformation progress. badly named I must admit :/ -
played_floor
- the ID of the played_floor on which this event happened -
video
- the 11-character ID of the video. See "videos" table. -
action
- same as above. a counter that counts up (+1) for every action that was taken by this character. Can be used to sort all actions chronologically. -
played_character
- the ID of the played_character from the above section to whom this event belongs. -
in_consequence_of
- reference to another gameplay event. Only used for:- Transformation Progress: what gameplay event triggered transformation progress? (will be "item collected" in most cases)
- Transformation Complete: what gameplay event triggered transformation? (will also be "item collected" in most cases)
- Lost the run: the "character died" event that triggered the loss
-
run_number
- same as above: a submission can have multiple runs. This is a counter that starts at 1 and is incremented for every run. Example:- Run 1: game_character = Isaac; run_number = 1
- Run 2: game_character = Maggie; run_number = 2
-
player
- Player 1 or 2. Used for runs including Jacob & Esau as well as possible future 2-player mode. -
floor_number
- same as above: starts at 1 and increments for every floor after that. can be used to sort events by floors in the order they appeared in the run. -
submission
- the ID of the submission this floor belongs to. -
was_rerolled
- true for items that were rerolled before being taken, touched or absorbed. false for everything else.
The very general "resource_one" and "resource_two" approach for all gameplay events might make it a little confusing about what is what.
Here is a list of how gameplay events correlate with resource_one and -two:
- Unspecified (unused, can be ignored)
-
Character Died
- ID of the enemy that killed the character
- -
-
Item Collected
- ID of the item that was collected
- ID of the item source that spawned the item (for example "ItemRoom" or "DemonJudgement")
-
Down to the next Floor!
- The floor on which we are from now on
- -
-
Bossfight
- ID of the boss
- -
-
Pill used
- ID of the Pill
- -
-
Tarot Card used
- ID of the Tarot Card
- -
-
Rune used
- ID of the Rune
- -
-
Trinket taken
- ID of the Trinket that was used from now on
- -
-
Floor was Cursed
- ID of the curse that was present on this floor
- -
-
Other Consumable used
- ID of the consumable
- -
-
Transformation Complete
- The ID of the item that triggered the transformation completion
- The ID of the transformation
-
Transformation Progress
- The ID of the item that contributed to the transformation progress
- The ID of the transformation
- How far into the transformation we are: for example if this is 2, this was guppy item number 2
- Last Floor (unused, ignore!)
-
Absorbed Item
- The ID of the item that was absorbed
- The ID of the thing that absorbed the item (for example the void or black rune)
-
Character Reroll
- The ID of the thing that rerolled the character (for example D100 or the 6-Room)
- -
-
Won the run
- The ID of the character who won the run (for example "Isaac" or "TheLost")
- The ID of the floor on which the run ended ("BasementOne" or "WombTwo")
-
Lost the run
- The ID of the character who died (for example "Isaac" or "TheLost")
- The ID of the floor on which the run ended ("BasementOne" or "WombTwo")
-
Item Touched (picked up and put down again right away)
- ID of the item that was touched
- ID of the item source that spawned the item (for example "ItemRoom" or "DemonJudgement")
-
Starting Trinket
- ID of the trinket with which the character started
- -
-
Clicker (changed character with the clicker)
- Is always "Clicker"
- The ID of the new character
-
Reroll Transform (transformation that happened after rerolling the character)
- ID of the thing that rerolled the character
- ID of the transformation that took place
-
Respawn (respawning after death due to extra lives)
- ID of the enemy that killed the character
- -
Query Examples
All events of an episode in chronological order
select * from gameplay_events
where video = 'c5PLC6nmOO4'
and latest = true
order by run_number, action;
The events from the first floors of all 3 runs from the 'Trainwreck' episode, in chronological order
select * from gameplay_events
where video = 'JfDnrW-pnXU'
and latest = true
and floor_number = 1
order by run_number, action;
Count Bossfights ordered by encounters
select resource_one, count(resource_one) as encounters
from gameplay_events
where event_type = 4
and latest = true
group by resource_one
order by encounters desc;
Get all items that triggered a transformation:
select resource_one as item, resource_two as transformation
from gameplay_events
where event_type = 11
and latest = true;
...with their real names instead of their ID, retrieved by a join on isaac_resources:
select items.name, transformations.name
from gameplay_events
join isaac_resources as items on gameplay_events.resource_one = items.id
join isaac_resources as transformations on gameplay_events.resource_two = transformations.id
where event_type = 11
and latest = true;
Mom-kills on sunday releases: with a simple join on the videos table
select gameplay_events.resource_one, count(gameplay_events.resource_one) as sunday_encounters
from gameplay_events
join videos on gameplay_events.video = videos.id
where gameplay_events.event_type = 4
and gameplay_events.resource_one = 'Mom'
and gameplay_events.latest = true
and extract(DOW from videos.published) = 0
group by gameplay_events.resource_one;
Find all guppy-items that were collected after already being guppy:
resource_three counts the steps towards a transformation, so a simple check on > 3 is enough:
select * from gameplay_events
where event_type = 12
and resource_three > 3
and resource_two = 'Guppy'
and latest = true;
discussion_topics
Stores general themes, discussion topics and tangents NL went on.

id
- Primary key.video
- the 11-character youtube video IDtopic
- the tangent, topic or themesubmitted_at
- timestamp when it was submitted
quotes
Stores funny NL-quotes users submitted

id
- primary keyvideo
- the 11-character youtube video IDcontent
- the actual quote textat
- how many seconds into the video the quote starts. can be used to directly link to the quote on youtubesubmitted_at
- timestamp when the quote was submitted
Query Examples
Get all quotes for an episode
select * from quotes where video = 'c5PLC6nmOO4';
Search quotes containing the f-word
select * from quotes where lower(content) like('%fridge%');
Search quotes for videos that have "cat" in the title (by joining the video table)
select videos.title, quotes.content
from quotes
join videos on quotes.video = videos.id
where lower(videos.title) like ('%cat%');
thumbnails
Stores thumbnail info that was pulled from youtube. Not all that interesting.

id
- primary keyurl
- full URL to the thumbnail imagewidth
- image widthheight
- image heightvideo
- the 11-character youtube video ID to which this thumbnail belongs to
transformative_resources
This table is used internally to automatically determine transformation progress throughout a run.
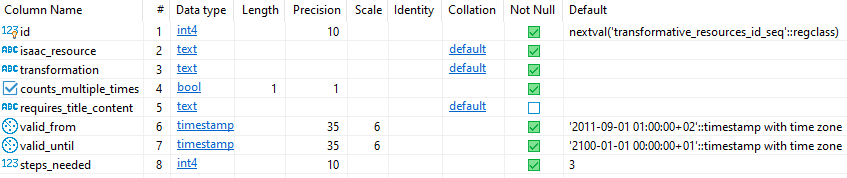
id
- primary keyisaac_resource
- the ID of the item, pill... that contributes to the transformation.transformation
- the ID of the transformation to which it contributes-
counts_multiple_times
- whether or not the item can contribute to the transformation progress multiple times. example: eating multiple "puberty"-pills will increase progress every time, but picking up "guppy's paw" multiple times will not! requires_title_content
- if the transformation progress only counts if the video title contains a specific set of characters, for example "community remix"valid_from
- video releasedate must be higher than this for it to countvalid_until
- video releasedate must be smaller that this for it to countsteps_needed
- how many things must be collected before transformation happens? Is 3 in all cases so far.